In a case I worked on, the error message “Invalid length for a Base-64 char array or string” has been logged by the application hundreds of times a day. There were no user side issues but the monitoring tool (DynaTrace) kept reporting this error.
Root Cause
In order to understand this error message, we should first understand the encoding from ASCII text to Base64. Long story short: Each character is represented by 8 bits in ASCII text. However, each of them is represented by 6 bits in Base64 string. Because of the conversion of characters from 8 bits to 6 bits, some padding characters (=) may need to be added to the end of the Base64 encoded string.
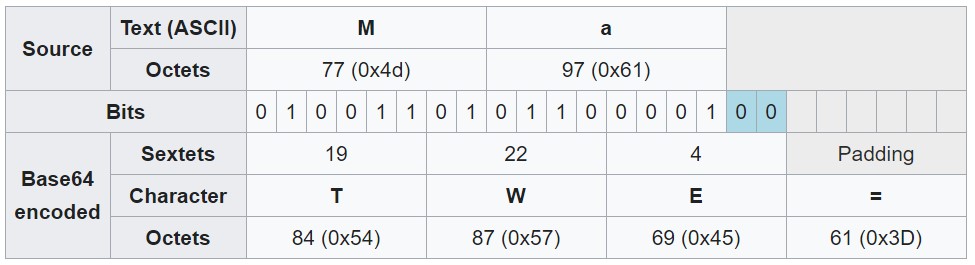
Wikipedia explains this conversation very well with examples: Base64
The application throwing this error is probably using Convert.FromBase64String
method to decode the Base64 string. If you log the encrypted string somewhere when this exception is thrown, you can find out what is wrong with this string. However, without looking at the string, I can tell that the issue is probably the length of the string as the error message points out. The length of the Base-64 string should be multiple of 4. If it’s not, the padding chracter (=) should be added to the end.
More information about the method: FromBase64String
Solution for the “Invalid length for a Base-64 char array or string” error
Implement the code block below to check the string length and add padding characters if needed:
int mod4 = encryptedtext.Length % 4;
if (mod4 > 0 )
{
encryptedtext += new string('=', 4 - mod4);
}
byte[] encryptedBytes = Convert.FromBase64String(encryptedtext);
You can also use HttpUtility.URLDecode to solve this issue.
Still Seeing the Error?
The second most common root cause of the Base-64 related errors is the space character in the encrypted string. In order to solve this issue, replace space with plus (+) sign.
Sample code:
string stringToDecrypt = CypherText.Replace(" ", "+");
byte[] inputByteArray =
Convert.FromBase64String(stringToDecrypt);
If you are receiving “400 Bad Request” message along with the Base64 error, check this post out: HTTP 400 Bad Request (Request header too long)