If you don’t want to store confidential information in your application’s code or configuration files, you can use Azure Key Vault. It’s FIPS 140-2 validated and easy to use.
I will explain the configuration required and share a sample code to retrieve secrets from Azure.
Get secret values from Azure Key Vault
First, create a Key Vault and Secret in your Azure account:
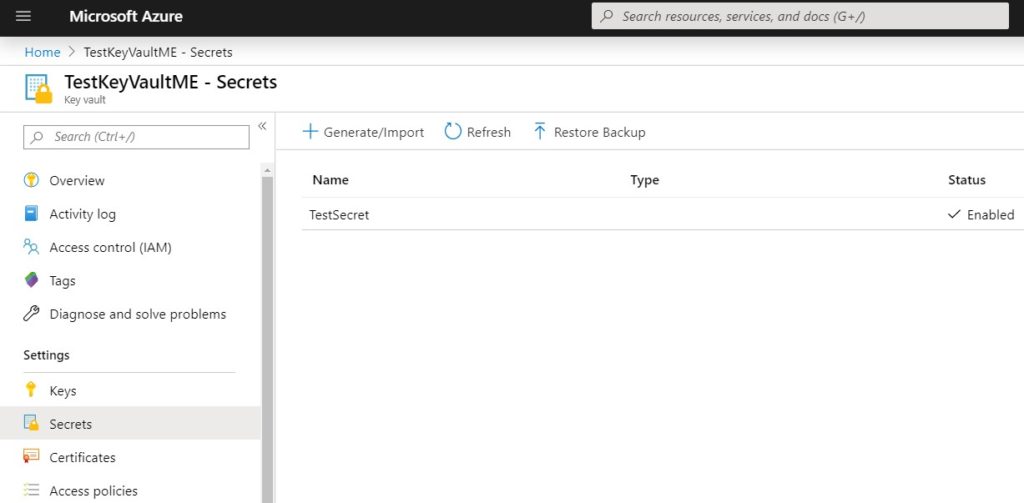
Go to “Access policies” and make sure the the account you will use has access to this Key Vault.
Once you complete settings in your Azure account, use the code below to retrieve the Secret you created. Make sure to change the Key Vault and Secret name in the following code.
Startup.cs:
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Configuration;
using System.Text;
namespace SampleApp
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; set; }
public void Configure(IApplicationBuilder app)
{
app.Run(async context =>
{
var recaptcha = Configuration.GetSection("TestSecret");
var encoding = new UTF8Encoding(encoderShouldEmitUTF8Identifier: false);
var document = string.Format(Markup.Text, Configuration["SecretName"], Configuration["Section:SecretName"], Configuration.GetSection("Section")["SecretName"]);
context.Response.ContentLength = encoding.GetByteCount(document);
context.Response.ContentType = "text/html";
await context.Response.WriteAsync(document);
});
}
}
}
Program.cs:
using System.Linq;
using System.Security.Cryptography.X509Certificates;
using Microsoft.AspNetCore;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Azure.KeyVault;
using Microsoft.Azure.Services.AppAuthentication;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.Configuration.AzureKeyVault;
namespace SampleApp
{
public static class Program
{
public static void Main(string[] args)
{
CreateWebHostBuilder(args).Build().Run();
}
public static IWebHostBuilder CreateWebHostBuilder(string[] args) =>
WebHost.CreateDefaultBuilder(args)
.ConfigureAppConfiguration((context, config) =>
{
if (context.HostingEnvironment.IsProduction())
{
var builtConfig = config.Build();
var azureServiceTokenProvider = new AzureServiceTokenProvider();
var keyVaultClient = new KeyVaultClient(
new KeyVaultClient.AuthenticationCallback(
azureServiceTokenProvider.KeyVaultTokenCallback));
config.AddAzureKeyVault(
$"https://{builtConfig["KeyVaultName"]}.vault.azure.net/",
keyVaultClient,
new DefaultKeyVaultSecretManager());
string secret = keyVaultClient.GetSecretAsync("https://testkeyvaultme.vault.azure.net/", "TestSecret").Result.Value;
}
})
.UseStartup<Startup>();
}
}
For more information: Configuration Provider in ASP.NET Core
If you are hosting this application in IIS and if you want to host it in multiple servers, you can use Azure file share. Check this post out for details: How to use Azure file share in IIS Shared Configuration?